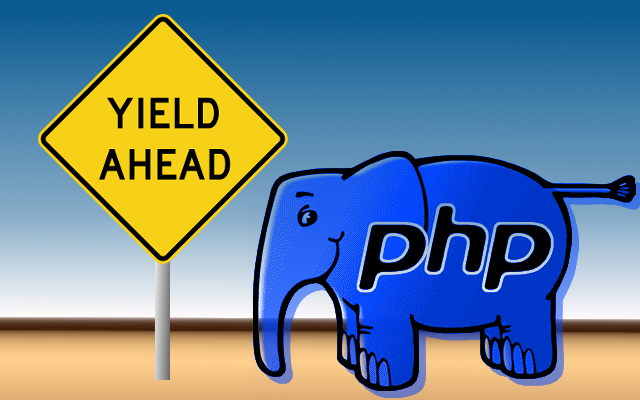
1.) Introduction
PHP Generators provide a simple way to iterate through data without needing to build an array in memory. They allow you to create iterators in a very simple way without implementing the Iterator
interface. Generators are particularly useful for handling large datasets or streams where holding the entire dataset in memory is impractical.
2.) What are Generators?
A generator allows you to iterate through a set of data without needing to build an array in memory. Instead, you use the yield
keyword to return values one at a time as needed.
2.i) Defining a Generator
To define a generator function, use the function
keyword followed by the yield
keyword inside the function body to return each value:
function myGenerator() {
yield 'value1';
yield 'value2';
yield 'value3';
}
// Using the generator
foreach (myGenerator() as $value) {
echo $value . PHP_EOL;
}
2.ii) Generator with a Loop
Generators can be particularly powerful when used with loops. Here’s an example of a generator function that yields values from a loop:
function numberGenerator($start, $end) {
for ($i = $start; $i <= $end; $i++) {
yield $i;
}
}
// Using the generator
foreach (numberGenerator(1, 5) as $number) {
echo $number . PHP_EOL;
}
3.) Advantages of Using Generators
Generators offer several advantages:
- Memory Efficiency: Generators yield values one at a time, so they can handle large datasets without consuming large amounts of memory.
- Simplicity: Generators provide a simple syntax for creating iterators, making code easier to read and maintain.
- Performance: Generators can improve performance by generating values on-the-fly instead of loading entire datasets into memory.
4.) Use Cases for Generators
Generators can be used in various scenarios where memory efficiency and simplicity are important:
- Reading large files line by line
- Generating a large sequence of numbers
- Fetching large datasets from databases or APIs
5.) Advanced Generator Features
Generators in PHP come with additional features that can be leveraged for more complex use cases:
5.i) Sending Values to Generators
You can send values back to a generator using the send()
method:
function printer() {
$string = '';
while (true) {
$string = yield $string;
echo $string . PHP_EOL;
}
}
$printer = printer();
$printer->send('Hello');
$printer->send('World');
5.ii) Generator Delegation
Generators can delegate to another generator using the yield from
syntax:
function subGenerator() {
yield 'Sub1';
yield 'Sub2';
}
function mainGenerator() {
yield 'Main1';
yield from subGenerator();
yield 'Main2';
}
foreach (mainGenerator() as $value) {
echo $value . PHP_EOL;
}
6.) Conclusion
PHP Generators provide a powerful tool for creating memory-efficient, simple iterators. By using the yield
keyword, developers can handle large datasets and streams more effectively. Generators are particularly useful in scenarios where loading the entire dataset into memory is impractical or inefficient. With their simple syntax and advanced features, generators can significantly improve both the performance and readability of your code.