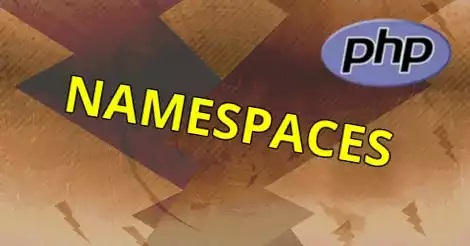
Overview
Namespaces in PHP are a way to encapsulate and organize code by grouping related classes, interfaces, functions, and constants. They help prevent name collisions and make code more modular and maintainable, especially in large applications or when integrating third-party libraries.
Namespaces provide a way to avoid name conflicts in large codebases by isolating the scope
1.) Introduction
Namespaces in PHP are a way to encapsulate and organize code by grouping related classes, interfaces, functions, and constants. They help prevent name collisions and make code more modular and maintainable, especially in large applications or when integrating third-party libraries.
2.) Overview of Namespaces
Namespaces provide a way to avoid name conflicts in large codebases by isolating the scope of identifiers. This is particularly useful when different parts of an application or multiple libraries use the same class, function, or constant names.
2.i) Defining a Namespace
To define a namespace in PHP, use the namespace
keyword at the top of your PHP file. All code within this file will belong to the specified namespace.
namespace MyApp\Utils;
class Helper {
public static function greet() {
return "Hello from MyApp\\Utils\\Helper!";
}
}
2.ii) Using Namespaces
To use namespaced code, you can either use the fully qualified name or employ the use
keyword to import the namespace.
// Using fully qualified name
echo \MyApp\Utils\Helper::greet();
// Using the 'use' keyword
use MyApp\Utils\Helper;
echo Helper::greet();
3.) Benefits of Namespaces
Namespaces provide several benefits:
- Prevent name collisions in large applications.
- Organize code into logical groups.
- Improve code readability and maintainability.
4.) Nested Namespaces
Namespaces can be nested to create a hierarchy, allowing for even more organized code structures.
namespace MyApp\ModuleA\SubModule;
class Component {
public static function info() {
return "This is MyApp\\ModuleA\\SubModule\\Component.";
}
}
5.) Aliasing Namespaces
You can use the as
keyword to alias namespaces, making it easier to reference long namespaces.
use MyApp\ModuleA\SubModule\Component as SubModuleComponent;
echo SubModuleComponent::info();
6.) Conclusion
Namespaces are a powerful feature in PHP that help organize code and prevent name conflicts. By using namespaces, developers can write more modular, maintainable, and scalable applications. They are essential for managing large codebases and integrating multiple libraries.